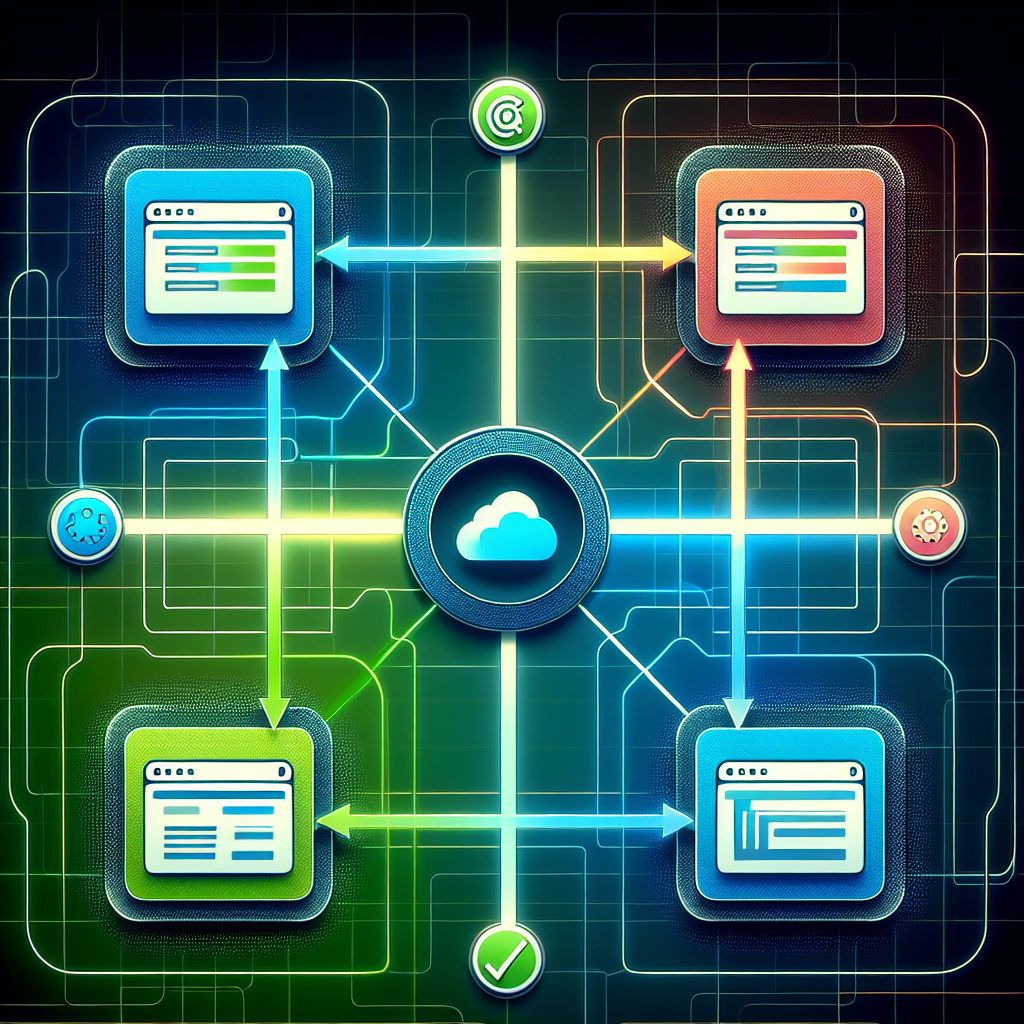
Say you are a very restrictive organization and have enabled an allowed list of domains your users can collaborate externally with. Did you know there are 4 different portals where you can add external domains? Did you know that none of them are synced/connected to each other? You can add external sharing domains to Entra ID Users, SharePoint, Teams, and Exchange Online. There is actually a fifth, in the B2B collaboration settings in Entra ID also, but that one we are skipping for today.
I have created a simple proof of concept that syncs the domains between the various portals. If you add a domain to Teams, it automatically adds itself to the three other portals. Before running this script, you need to add one domain to the allowed list for each of these portals, so all the correct settings are set up. Once again, this is a proof of concept. Even though it works, it could be made much more complex and advanced.
# Connect to Azure Account | |
Connect-AzAccount | |
# Connect to Microsoft Teams | |
Connect-MicrosoftTeams | |
# Connect to Exchange Online | |
Connect-ExchangeOnline | |
# Connect to SharePoint Online | |
$SPConnection = Connect-PnPOnline -Url https://alexholmeset-admin.sharepoint.com -Interactive | |
# Get access token for Microsoft Graph API | |
$authToken = Get-AzAccessToken -ResourceUrl "https://graph.microsoft.com" | |
# Set the header for the API requests | |
$Header = @{authorization = "Bearer " + $authToken.Token} | |
# Get the organization relationship in Exchange Online | |
$ExchangeOrganization = Get-OrganizationRelationship | |
$ExchangeOrganization = $ExchangeOrganization.DomainNames| Where-Object {$_ -notlike "*Calendar*"} | |
# Get the individual sharing policy in Exchange Online | |
$ExchangeInduvidual = Get-SharingPolicy | |
$ExchangeInduvidual = ($ExchangeInduvidual.Domains | Where-Object {$_ -like "*.*:*"}).split(":") | Where-Object {$_ -notlike "*Calendar*"} | |
# Get the Teams tenant federation configuration | |
$Teams = Get-CsTenantFederationConfiguration | |
$Teams = $Teams.AllowedDomains.alloweddomain.domain | |
# Get the tenant information in SharePoint | |
$SharePoint = Get-PNPTenant -connection $SPConnection | |
$SharePointPreSplit = $SharePoint.SharingAllowedDomainList | |
$SharePoint = ($SharePoint.SharingAllowedDomainList).split(" ") | |
$SharePointDomainlist = $SharePointPreSplit | |
# Get the Azure AD legacy policies | |
$AzureAD = (invoke-restmethod -Method get -Uri "https://graph.microsoft.com/beta/legacy/policies/" -Headers $Header -ContentType "application/json").value | |
$AzureADID = $AzureAD.id | |
$AzureADRequest = (((($AzureAD.definition).split('{"AllowedDomains":['))[1].split(']'))[0]).replace('"','\"') | |
$AzureAD = ($AzureAD.definition | convertfrom-json ).b2bmanagementpolicy.InvitationsAllowedAndBlockedDomainsPolicy.alloweddomains | |
# Set the sharing policy in Exchange Online | |
# Set-SharingPolicy -Identity Contoso.com -Domains "contoso.com: CalendarSharingFreeBusySimple" | |
# Print the information gathered | |
"Exchange Online Organization:" | |
$ExchangeOrganization | |
"" | |
"Exchange Online Induvidual:" | |
$ExchangeInduvidual | |
"" | |
"Teams:" | |
$Teams | |
"" | |
"SharePoint:" | |
$SharePoint | |
"" | |
"Azure AD:" | |
$AzureAD | |
# Combine all the domains | |
$Domains = @() | |
$Domains += $ExchangeOrganization | |
$Domains += $ExchangeInduvidual | |
$Domains += $Teams | |
$Domains += $SharePoint | |
$Domains += $AzureAD | |
$Domains = ($Domains | Select-Object -Unique | Group-Object).Name | |
$AzureADList = @() | |
# Check each domain and add to the respective allowed domains list if not already present | |
foreach ($Domain in $Domains){ | |
if (-not ($ExchangeOrganization -contains $Domain)) { | |
New-OrganizationRelationship -Name "$Domain" -DomainNames "$Domain" -FreeBusyAccessEnabled $true -FreeBusyAccessLevel AvailabilityOnly -TargetAutodiscoverEpr "https://autodiscover-s.outlook.com/autodiscover/autodiscover.svc/WSSecurity" -TargetApplicationUri "outlook.com" | |
} | |
if (-not ($ExchangeInduvidual -contains $Domain)) { | |
$domaininduvidual = $Domain+": CalendarSharingFreeBusySimple" | |
# New-SharingPolicy -Name $Domain -Domains "$domaininduvidual" | |
} | |
if (-not ($Teams -contains $Domain)) { | |
$list = New-Object Collections.Generic.List[String] | |
$list.add("$Domain") | |
Set-CsTenantFederationConfiguration -AllowedDomainsAsAList @{Add=$list} | |
} | |
if (-not ($SharePoint -contains $Domain)) { | |
$SharePointDomainlist += " $Domain" | |
} | |
if (-not ($AzureAD -contains $Domain)) { | |
$AzureADList += ',\"'+$domain+'\"' | |
} | |
} | |
# Update the Azure AD legacy policies with the new allowed domains | |
If($AzureADList){ | |
$AzureADList = $AzureADList -join "" | |
$Policy = @" | |
{ | |
"definition": [ | |
"{\"B2BManagementPolicy\":{\"InvitationsAllowedAndBlockedDomainsPolicy\":{\"AllowedDomains\":[$($AzureADRequest+$AzureADList)]},\"AutoRedeemPolicy\":{\"AdminConsentedForUsersIntoTenantIds\":[],\"NoAADConsentForUsersFromTenantsIds\":[]}}}" | |
] | |
} | |
"@ | |
invoke-restmethod -Method patch -Uri "https://graph.microsoft.com/beta/legacy/policies/$AzureADID" -Headers $Header -ContentType "application/json" -Body $Policy | |
} | |
# Update the SharePoint allowed domains list | |
$domainlist = $SharePointPreSplit+" $Domain" | |
Set-PnPTenant -SharingCapability ExistingExternalUserSharingOnly -SharingDomainRestrictionMode AllowList -SharingAllowedDomainList "$SharePointDomainlist" -connection $SPConnection |